Command line Argument in Java
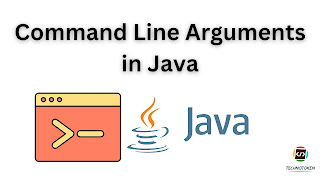
What are Command Line Arguments in Java?
A Java command-line parameter is an argument that is passed when the Java application is executed. The parameters given from the terminal can be accepted and utilized as input in the java application via the command line. Users can supply parameters to the main() function during execution, bypassing the command-line arguments.
Also, the command-line arguments are separated by spaces by default. However, they can also be enclosed in quotes to be considered as a single argument. So the parameters must be supplied as space-separated values. As command-line parameters, we can send texts as well as primitive data types (int, double, float, char, and so on). These parameters are converted into a string array and sent as a string array argument to the main() method.
Syntax
public class MyClass {
public static void main(String[] args) {
// Retrieve the command line arguments
String arg1 = args[0];
String arg2 = args[1];
// ...
// Use the command line arguments in your program
// ...
}
}
To use command line arguments in Java, you need to declare the main method with a parameter of type String[]. This parameter is an array of strings that represents the command line arguments passed to the program.
You can then access the command line arguments using the args array. The first argument is stored in args[0], the second argument is stored in args[1], and so on.
Once you have retrieved the command line arguments, you can use them in your program as needed. This could involve performing calculations, reading files, connecting to databases, or any other task that your program needs to perform based on the input provided by the user.
How are Command Line Arguments Used in Java?
Command line arguments can be used to modify the behavior of a Java program. For example, a Java program that calculates the square root of a number can be modified to accept a command line argument that specifies the number whose square root is to be calculated. Similarly, a Java program that reads data from a file can be modified to accept a command line argument that specifies the name of the file to be read.
Command line arguments can also be used to provide information that a Java program needs to run. For example, a Java program that connects to a database can be modified to accept command line arguments that specify the database URL, username, and password.
Examples
Let's take a look at some examples of using command line arguments in Java.
Example 1: A Java program that calculates the sum of two numbers
public class AddTwoNumbers {
public static void main(String[] args) {
int num1 = Integer.parseInt(args[0]);
int num2 = Integer.parseInt(args[1]);
int sum = num1 + num2;
System.out.println("The sum of " + num1 + " and " + num2 + " is " + sum);
}
}
In this example, we are accepting two command line arguments, converting them to integers using the parseInt method of the Integer class, and then adding them to calculate the sum. The result is then displayed on the console.
To run this program, you would need to open a command prompt or terminal window and navigate to the directory where the program is located. Then, you would type the following command:
java AddTwoNumbers 10 20
This would pass the values 10 and 20 as command line arguments to the program, and the output would be:
The sum of 10 and 20 is 30
Example 2: A Java program that reads data from a file
import java.io.*;
public class ReadFile {
public static void main(String[] args) {
try {
File file = new File(args[0]);
BufferedReader br = new BufferedReader(new FileReader(file));
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
br.close();
} catch (IOException e) {
System.out.println("Error reading file: " + e.getMessage());
}
}
}
To run this program, you would need to open a command prompt or terminal window and navigate to the directory where the program is located. Then, you would type the following command:
java ReadFile filename.txt
This would pass the name of the file filename.txt as a command line argument to the program, and the contents of the file would be displayed on the console.
Frequently Asked Questions (FAQ)
How to find length of command line arguments in java?
To find the length of command line arguments in Java, you can use the length property of the args array. This property returns the number of elements in the array, which is equal to the number of command line arguments passed to the program.
Here's an example code snippet that demonstrates how to find the length of command line arguments in Java:
public class MyClass {
public static void main(String[] args) {
int numArgs = args.length;
System.out.println("Number of command line arguments: " + numArgs);
}
}
In this example, we are using the length property of the args array to find the number of command line arguments passed to the program. We are then storing this value in the variable numArgs, and printing it to the console using System.out.println().
To run this program and pass command line arguments to it, you can open a command prompt or terminal window and navigate to the directory where the program is located. Then, you can type the following command:
java MyClass arg1 arg2 arg3
This command passes three command line arguments (arg1, arg2, and arg3) to the program. The program will then print the number of command line arguments, which in this case will be 3.
By using the length property of the args array, you can easily find the number of command line arguments passed to your Java program and use it to control the flow of your program accordingly.
How to parse command line arguments in java?
Parsing command line arguments in Java can be done using the args array that is passed to the main() method of your Java program. The args array contains all the command line arguments passed to your program, separated by spaces.
To parse command line arguments, you can iterate through the args array using a loop and use conditional statements to check the value of each argument.
Here's an example code snippet that demonstrates how to parse command line arguments in Java:
public class MyClass {
public static void main(String[] args) {
String inputFile = null;
String outputFile = null;
boolean verbose = false;
for (int i = 0; i < args.length; i++) {
String arg = args[i];
switch (arg) {
case "-input":
if (i + 1 < args.length) {
inputFile = args[i+1];
i++; // Move to the next argument
} else {
System.err.println("Missing argument for -input");
System.exit(1);
}
break;
case "-output":
if (i + 1 < args.length) {
outputFile = args[i+1];
i++; // Move to the next argument
} else {
System.err.println("Missing argument for -output");
System.exit(1);
}
break;
case "-verbose":
verbose = true;
break;
default:
System.err.println("Invalid argument: " + arg);
System.exit(1);
}
}
// Do something with the input, output, and verbose values
}
}
What is args in java?
In Java, args is a command line argument that is used to pass values to your Java program when it is executed from the command line. The args variable is an array of strings that contains all the command line arguments passed to your program.
When you run a Java program from the command line, you can pass command line arguments to the program by including them after the program name. For example, if you have a Java program called MyProgram and you want to pass two command line arguments to it, you would run the program with a command like this:
java MyProgram arg1 arg2
public class MyClass {
public static void main(String[] args) {
for (int i = 0; i < args.length; i++) {
System.out.println("Argument " + i + ": " + args[i]);
}
}
}
In this example, we are using a for loop to iterate through the args array and print out the value of each command line argument to the console. When you run this program with the command java MyClass arg1 arg2 arg3, it will print the following output:
Argument 0: arg1 Argument 1: arg2 Argument 2: arg3
What is string args in main method?
In Java, String args[] is a parameter that is passed to the main() method of your program. This parameter is an array of strings that represents the command line arguments passed to your program when it is executed.
When you run a Java program from the command line, you can pass command line arguments to the program by including them after the program name. For example, if you have a Java program called MyProgram and you want to pass two command line arguments to it, you would run the program with a command like this:
java MyProgram arg1 arg2
public class MyClass {
public static void main(String[] args) {
for (int i = 0; i < args.length; i++) {
System.out.println("Argument " + i + ": " + args[i]);
}
}
}
Argument 0: arg1 Argument 1: arg2 Argument 2: arg3
Thanks for Scrolling… 😄
Today we were able to learn a little bit about Command line Argument in Java. We also deep dived in what command line argument in java are used for with various frequently asked questions about it. If you want us to write a more detailed post on this topic or have any particular queries regarding this post you can always comment or feel free to mail us at our official mail. Also, make sure to follow us on Instagram and Facebook or subscribe to our newsletter to receive Latest Updates first.
Peace!!!
No comments
If you have any doubts, please let us Know.