For Loop In C
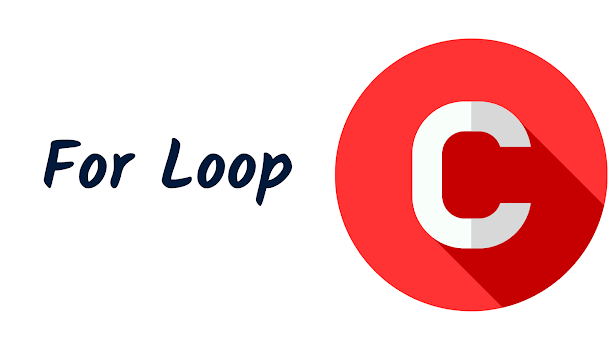
For Loop In C
A for loop is a control flow statement in programming that allows you to iterate over a block of code a specific number of times. It is a popular programming construct that is widely used across different programming languages.
The basic structure of a for loop consists of an initialization expression, a conditional expression, and an update expression. The initialization expression is executed once at the beginning of the loop, the conditional expression is evaluated at the beginning of each iteration to determine if the loop should continue or terminate, and the update expression is executed at the end of each iteration.
For loops are commonly used when you need to perform a repetitive task for a specific number of times. For example, you might use a for loop to print out the numbers from 1 to 10, or to calculate the sum of the first 100 integers.
One of the main benefits of using a for loop is that it allows you to write concise, efficient code. Instead of repeating the same code over and over, you can use a for loop to encapsulate the repetitive logic, making your code more readable and maintainable.
In addition to the basic structure, for loops can be extended with additional keywords such as break and continue to provide additional control over the loop's behavior. For example, you might use the break keyword to exit a loop early if a specific condition is met, or the continue keyword to skip over specific iterations of the loop.
Overall, for loops are a fundamental building block of programming and are essential for writing efficient, maintainable code. By mastering the for loop, you will be able to write more complex programs with less code, making you a more effective and productive programmer.
Syntax of For Loop
// Syntax of For loop in C Programming Language
#include
for (initialization; condition; increment/decrement) {
// code to be executed
}
How does a for loop work in C?
A for loop in C works by first initializing a counter variable, checking a condition to see if it is still true, and then executing a block of code. The loop continues until the condition is false. After each iteration of the loop, the counter variable is incremented (or decremented) based on the increment statement provided.
Example of For Loop In C
#include <stdio.h> int main() { for (int i = 1; i <= 10; i++) { printf("%d ", i); } return 0; }
#include <stdio.h> int main() { int sum = 0; for (int i = 2; i <= 100; i += 2) { sum += i; } printf("Sum of even numbers from 1 to 100: %d", sum); return 0; }
Flowchart of For Loop In C
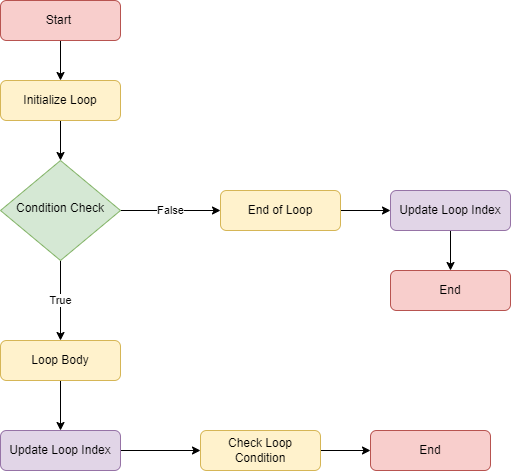
Frequently Asked Questions (FAQ)
What are the best practices for using for loops in C?
For loops are a crucial part of programming in C, allowing developers to iterate over a set of values and perform actions on each iteration. However, the effectiveness of for loops depends on how well they are constructed.
Here are some best practices for using for loops in C, including ways to enhance code efficiency, readability, and avoid common errors:
- Initialize loop counter outside the loop: It's always better to initialize the loop counter before the loop instead of doing it inside the loop, as it can lead to unnecessary overhead.
- Use meaningful loop counter names: Using meaningful loop counter names can make your code more readable and understandable.
- Use braces {} for multiple lines of code: If you have multiple lines of code in your loop, it's better to use braces to enclose them. This helps to avoid confusion and makes your code more readable.
- Use a break statement to exit the loop: If you need to exit a loop before it has completed all of its iterations, use a break statement instead of relying on the loop's condition to become false.
- Use a continue statement to skip an iteration: If you need to skip an iteration of a loop, use the continue statement to move to the next iteration.
- Use pre-increment or pre-decrement operators for the loop counter: Pre-increment or pre-decrement operators (++i or --i) are generally more efficient than post-increment or post-decrement operators (i++ or i--).
- Avoid infinite loops: Make sure your loop has a condition that will eventually become false, or use a break statement to exit the loop.
- Use a nested for loop when necessary: If you need to iterate over a multidimensional array or perform a nested iteration, use a nested for loop.
By following these best practices, you can write efficient, readable, and bug-free code using for loops in C.
How to use a for loop to print a pattern of asterisks in C?
To use a for loop to print a pattern of asterisks, you can start by defining the number of rows and columns in the pattern. Then, you can use nested for loops to print the asterisks in the desired pattern.
Here is an example code snippet for it:
#include <stdio.h> int main() { int rows; printf("Enter the number of rows: "); scanf("%d", &rows); for (int i = 1; i <= rows; i++) { for (int j = 1; j <= i; j++) { printf("* "); } printf("\n"); } return 0; }
Can I use a for loop to iterate over an array in C?
Yes, a for loop is commonly used to iterate over an array in C. You can use the length of the array to determine the number of times to execute the loop.
Here's a sample code snippet to demonstrate how to use a for loop to iterate over an array:
#include <stdio.h> int main() { int arr[5] = {10, 20, 30, 40, 50}; int i; for(i = 0; i < 5; i++) { printf("Value of arr[%d] = %d\n", i, arr[i]); } return 0; }
How do I break out of a for loop in C?
To break out of a for loop in C, you can use the break statement. The break statement will immediately exit the loop and continue with the next line of code after the loop.
In C programming, there are a few ways to break out of a for loop, depending on the specific scenario.
Here's are some example code snippets that demonstrates three different approaches:
Approach 1: Breaking out of a for loop with a condition
This approach uses a conditional statement (if) inside the for loop to check if a certain condition is true.
#include <stdio.h> int main() { int i; // Breaking out of a for loop with a conditionfor (i = 0; i < 10; i++) { if (i == 5) { break; // This will immediately exit the loop when i == 5 } printf("%d ", i); } printf("\n");return 0; }
In this example, when the condition is true i.e. when i == 5, the break statement is executed, which immediately exits the loop.
This approach is very useful when you want to break out of a loop based on a specific condition that can be checked within the loop body.
#include <stdio.h> int main() { int i; // Breaking out of a for loop with a goto statementfor (i = 0; i < 10; i++) { if (i == 5) { goto end_loop; // This will jump to the end of the loop when i == 5 } printf("%d ", i); } printf("\n"); end_loop: return 0; }
In this example, when the condition is true (again, when i == 5), the goto statement is executed, which jumps to the end_loop label and continues executing from there.
This approach is generally considered less readable and more error-prone than using a conditional statement, but it can be useful in certain situations (e.g. breaking out of multiple nested loops at once).
#include <stdio.h> int main() { int i; // Breaking out of nested for loops with a labeled break statementfor (i = 0; i < 10; i++) { for (int j = 0; j < 5; j++) { if (i == 5 && j == 3) { goto end_nested_loops; // This will jump to the end of both loops when i == 5 and j == 3 } printf("(%d, %d) ", i, j); } printf("\n"); } end_nested_loops: return 0; }
In this example, there are two nested for loops, and we want to break out of both loops when i == 5 and j == 3.
To do this, we define a label (end_nested_loops) before the outer loop and use a labeled break statement to jump to that label when the condition is true.
This approach can be useful when you have multiple nested loops and need to break out of all of them based on a specific condition.
How do I continue to the next iteration of a for loop in C?
To continue to the next iteration of a for loop in C, you can use the continue statement. The continue statement will immediately skip the remaining code in the loop and start the next iteration.
Here's an example of how you can use the continue statement in a for loop to skip over a specific iteration and continue with the next iteration:
#include <stdio.h> int main() { int i; for (i = 1; i <= 10; i++) { if (i == 5) { continue; // skip over this iteration } printf("%d\n", i); } return 0; }
Output
1
2
3
4
6
7
8
9
10
Conclusion
In conclusion, a for loop in C is a powerful programming construct that enables users to iterate over a block of code multiple times. Its simple syntax makes it easy to use and understand, and it can be a valuable tool in many different programming scenarios.
By using a for loop, programmers can efficiently manipulate data, perform repetitive tasks, and automate complex processes. Additionally, the flexibility of the loop's syntax allows for a wide range of customization and fine-tuning to meet specific programming needs.
Thanks for Scrolling… 😄
If you want us to write a more detailed post on this topic or have any particular queries regarding this post you can always comment or feel free to mail us at our official mail. Also, make sure to follow us on Instagram and Facebook or subscribe to our newsletter to receive Latest Updates first.
Peace!!!
No comments
If you have any doubts, please let us Know.