Structure vs. Union in C: What's the Difference and Why Does It Matter?
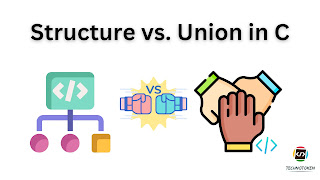
C programming language provides various data structures to organize and store data efficiently. Two such data structures are structure and union. While they might seem similar at first glance, they have significant differences that make them suitable for different use cases. In this article, we will explore the key differences between structure and union in C programming, and why it matters.
According to a recent study, C programming is one of the most popular programming languages, with a market share of over 15%. Therefore, understanding the differences between structure and union in C programming is crucial for developers who want to write efficient and effective code. By the end of this article, you will have a clear understanding of the key differences between structure and union in C programming, and when to use each.
Structure Vs Union
A structure is a collection of variables of different data types grouped together under a single name. Each of the variables within the structure is given a unique name and can be accessed individually. The variables within a structure can be of any data type, including arrays and other structures.
On the other hand, a union is a special data type that allows the storage of different data types in the same memory location. In a union, each member shares the same memory space, which means that only one of the members can be active at any given time. In simpler terms, a union is like a structure, but it only occupies enough memory to hold the largest member within it.
Key Differences
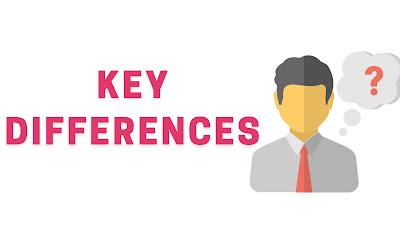
1. Memory allocation
The most significant difference between structure and union is the way in which they allocate memory. In a structure, memory is allocated for each member variable, and the total size of the structure is the sum of the sizes of its members. This means that each member of a structure has its own unique memory address.
In contrast, a union only allocates memory for the largest member variable. This means that all members of a union share the same memory space, and only one member can be active at a time. The size of a union is determined by the size of its largest member variable.
2. Accessing Members
The way in which members are accessed in a structure and a union is also different. In a structure, each member has a unique name, and it can be accessed using the dot (.) operator.
For example: If we have a structure called "employee" with members "name" and "age", we can access them using the following syntax:
struct employee emp;
emp.name = "John Doe";
emp.age = 30;
In a union, all members share the same memory space, and we can only access one member at a time. We use the same syntax as a structure to access a member of a union. However, we can only access the member that is currently active.
For example:
union data {
int i;
float f;
} d;
d.i = 10;
printf("%d", d.i); // output 10
d.f = 3.14;
printf("%f", d.f); // output 3.14
printf("%d", d.i); // output garbage value
In this example, we first assigned a value to the integer member "i". We then access it and print it out. Next, we assign a value to the float member "f", and print it out. Finally, we try to access the integer member "i" again, but we get a garbage value because the float member "f" is now active, and it has overwritten the value of "i".
3. Initialization
The way in which we initialize a structure and a union is also different. In a structure, we can initialize all members at once, or we can initialize each member separately.
For example:
struct employee {
char name[20];
int age;
};
// Initializing all members at once
struct employee emp = {"John Doe", 30};
// Initializing members separately
struct employee emp;
strcpy(emp.name, "John Doe");
In a union, we can only initialize one member at a time. For example:
union data {
int i;
float f;
} d = {.f = 3.14};
In conclusion, structure and union are both important concepts in C and C++ programming languages, but they are used in different ways. Understanding the key differences between structure and union is crucial for writing efficient and effective code.
Why Does It Matter?
Understanding the uses of structures and unions in C programming is important for creating efficient and effective code. By using these data structures appropriately, developers can create programs that are easier to read, maintain, and debug.
Structure Uses
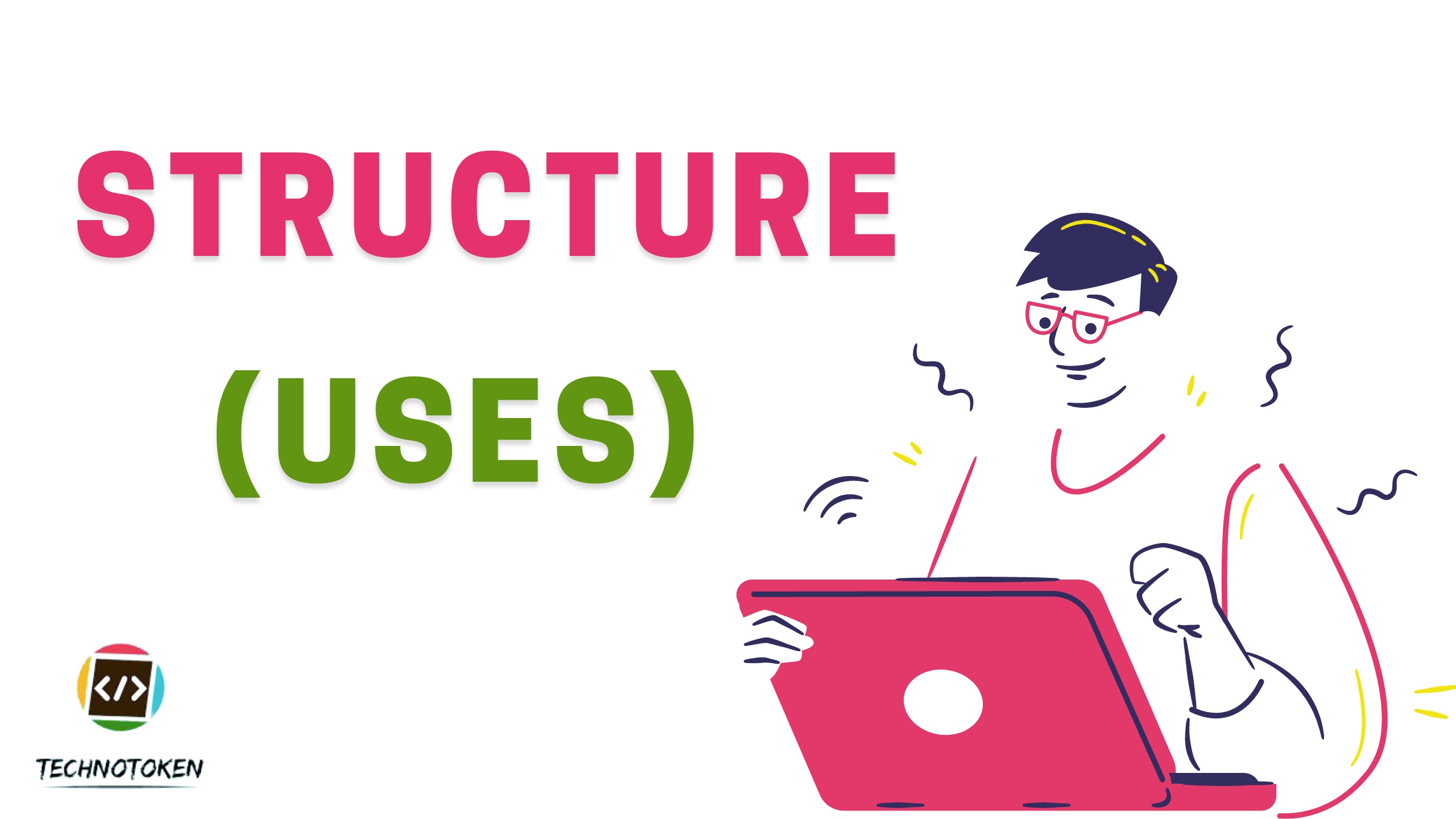
As we have already know that structure is a user-defined data type that groups related data of different types into a single unit. It easily allows the programmers to create a complex data type by combining the simple data types.
Here are some common uses of structures in C programming:
1. To represent complex real-world entities:
Structures can be used to represent complex entities such as a person or a car that have multiple attributes.
For example:
struct person {
char name[50];
int age;
float height;
float weight;
};
2. To pass multiple arguments to functions:
Structures can be used to pass multiple arguments to functions instead of passing them separately.
For example:
void print_person(struct person p) {
printf("Name: %s\nAge: %d\nHeight: %f\nWeight: %f\n", p.name, p.age, p.height, p.weight);
}
int main() {
struct person john = {"John", 25, 6.0, 180.0};
print_person(john);
return 0;
}
Union Uses
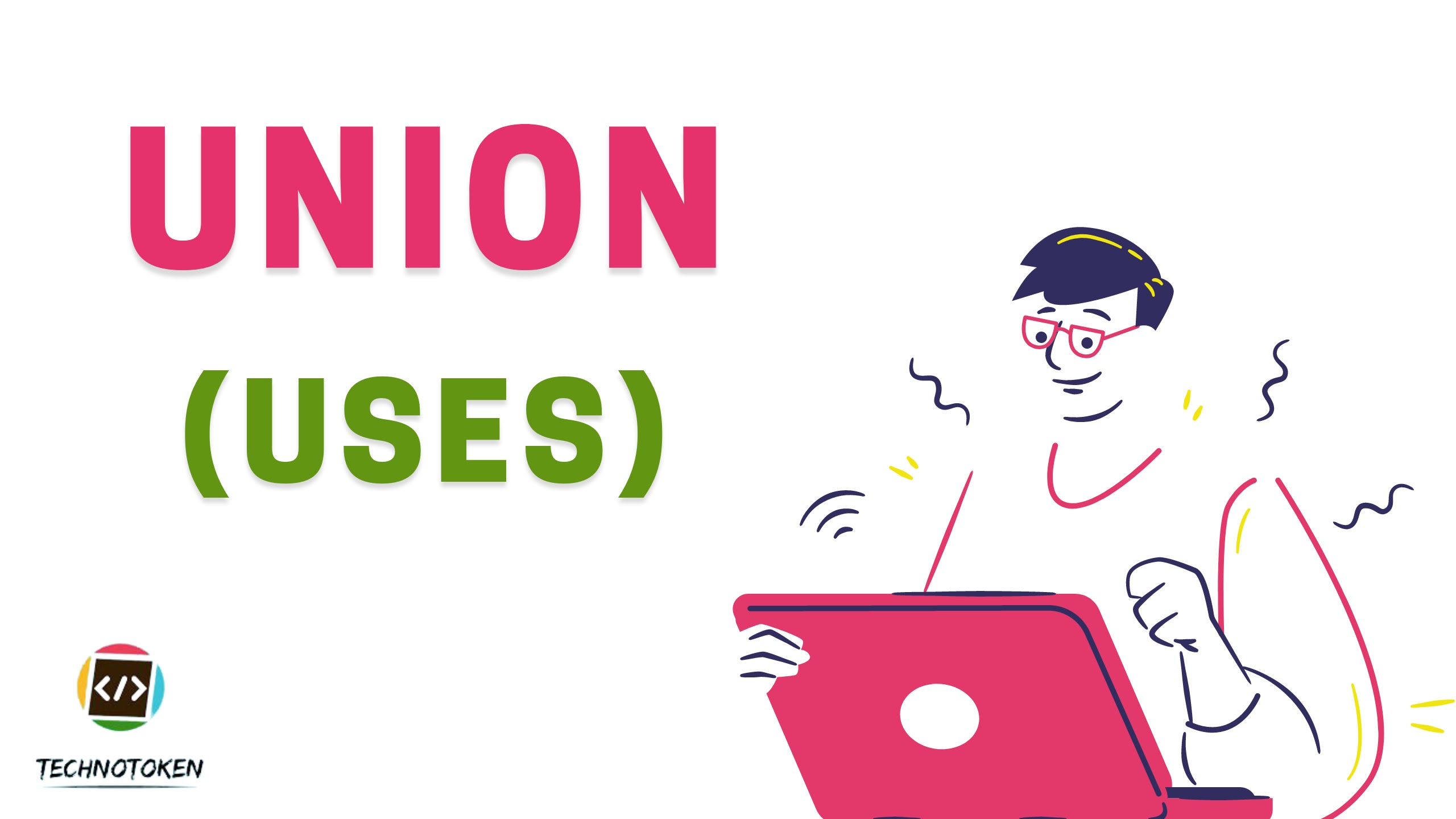
A union on the other hand is a user-defined data type that allows the programmer to store different types of data in the same memory location. It often comes useful in situations where memory is limited, as it allows for efficient use of memory.
Here are some common uses of unions in C programming:
1. To store different types of data in the same memory location:
Unions can be used to store different types of data in the same memory location, which can be useful in situations where you need to save memory.
For example:
union data {
int i;
float f;
char str[20];
};
int main() {
union data d;
d.i = 10;
printf("Value of d.i: %d\n", d.i);
d.f = 3.14;
printf("Value of d.f: %f\n", d.f);
strcpy(d.str, "Hello");
printf("Value of d.str: %s\n", d.str);
return 0;
}
2. To work with binary data:
Unions can be used to work with binary data, where you need to access individual bytes of a data type.
For example:
union bin_data {
int i;
unsigned char byte[4];
};
int main() {
union bin_data d;
d.i = 1000;
printf("Byte 1: %d\n", d.byte[0]);
printf("Byte 2: %d\n", d.byte[1]);
printf("Byte 3: %d\n", d.byte[2]);
printf("Byte 4: %d\n", d.byte[3]);
return 0;
}
Frequently Asked Questions (FAQ)
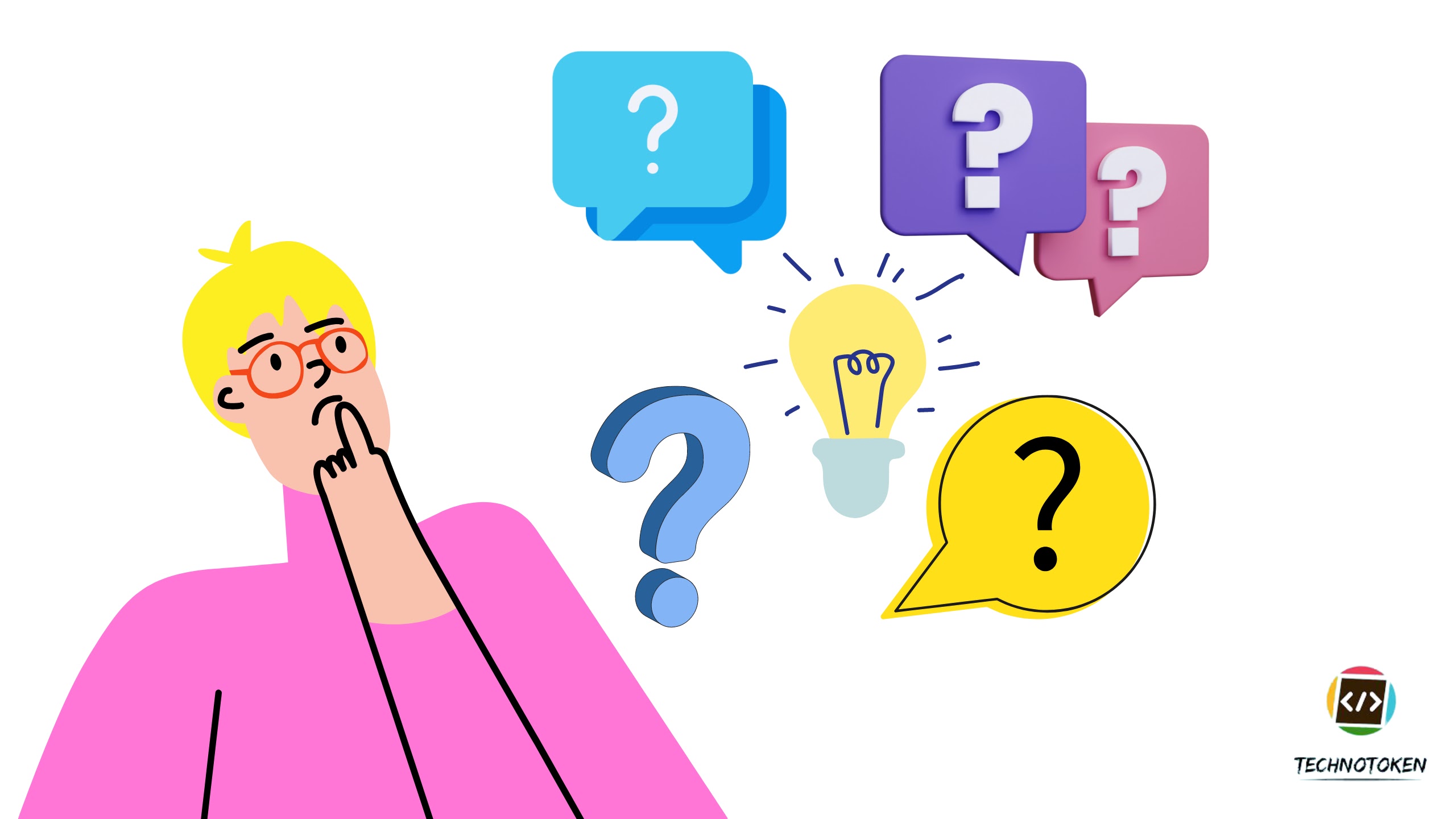
Can a structure contain a union in C?
Yes, a structure can contain a union in C. This is called a nested union, and it allows you to store different types of data in a more complex data structure. When you define a nested union within a structure, you can access the union members using the dot notation after accessing the structure members.
What are some common use cases for structures and unions in C programming?
Structures are commonly used to define complex data structures such as linked lists, trees, and graphs. They are also used to define user-defined data types in C. Unions, on the other hand, are useful when you need to conserve memory or when you need to store different types of data in a single memory location. For example, they are used in compilers to store the different types of tokens that can be found in a program.
What is the advantage of using a union instead of a structure in C?
The advantage of using a union instead of a structure in C is that a union can save memory by allowing different types of data to share the same memory location. This can be useful in situations where memory is limited or when you need to optimize performance. However, it's important to use unions carefully because they can lead to unpredictable behavior if you're not careful.
When should I use a structure instead of a union in C?
You should use a structure when you need to store multiple pieces of related data and access them individually. For example, if you want to store the information about a person, such as their name, age, and address, you would use a structure. On the other hand, you should use a union when you need to store different types of data in the same memory location. For example, if you have a variable that can hold an integer or a floating-point number, you would use a union.
How can I declare a union or structure variable in C?
To declare a structure variable in C, you need to define the structure using the "struct" keyword and then declare a variable of that type.
For example:
struct person {
char name[50];
int age;
float height;
};
struct person myPerson;
To declare a union variable in C, you need to define the union using the "union" keyword and then declare a variable of that type.
For example:
union myUnion {
int i;
float f;
char c;
};
union myUnion myVar;
These declarations create a variable of the specified type and allocate memory for it, ready to be used in your program.
What is the difference between a union and an enum in C?
A union and an enum are two different types of data structures in C. A union is a single variable that can hold different types of data at different times, while an enum is a set of named values that represent a set of related options or choices. For example, you might use a union to store either an integer or a floating-point number, while you might use an enum to represent the different colors of a traffic light.
How do I initialize a structure or union in C?
To initialize a structure or union in C, you can use the curly braces syntax to set the values of its members.
For example:
struct person {
char name[50];
int age;
float height;
};
struct person myPerson = {"John Doe", 30, 1.8};
union myUnion {
int i;
float f;
char c;
};
union myUnion myVar = {5}; // initializes the first member (i) to 5
This sets the initial values of the members of the structure or union, making it ready to use in your program.
Thanks for Scrolling… 😄
Today we were able to learn a little bit about the differences between a structure and a union in C/C++. If you want us to write a more detailed post on this topic or have any particular queries regarding this post you can always comment or feel free to mail us at our official mail. Also, make sure to follow us on Instagram and Facebook or subscribe to our newsletter to receive Latest Updates first.
Peace!!!
No comments
If you have any doubts, please let us Know.